#shorts
Multiple variable assignment - In Python, multiple variable assignment allows you to assign values to multiple variables in one line of code. This can be useful for assigning multiple values to variables that have a direct relationship with each other. Here's an example:
Ex - a, b = 10, 20
This line of code assigns the value 10 to the variable a and the value 20 to the variable b. You can also use tuple unpacking to assign values from a tuple to multiple variables.
Delete items in a list - Using the del statement: You can use the del statement to remove items from the list. Here's an example:
list = [1,2,3,4,5]
del list[1::2]
print(list)
Swapping -
Swapping in Python refers to exchanging the values of two or more variables. This can be done in several ways:
1. Using a temporary variable: You can use a temporary variable to store the value of one of the variables and then assign the value of the other variable to it. Here's an example:
a = 10
b = 20
temp = a
a = b
b = temp
print(a, b) # Output: 20 10
2. Using tuple unpacking: You can use tuple unpacking to swap the values of two variables. Here's an example:
a = "Hello"
b = "World"
a, b = b, a
print(a, b)
3. Using arithmetic operations: You can also use arithmetic operations to swap the values of two variables. Here's an example:
a = 10
b = 20
a = a + b
b = a - b
a = a - b
print(a, b) # Output: 20 10
▬▬▬▬▬▬ ⭐️ Time Stamps ⭐️ ▬▬▬▬▬▬
0:00 Multiple variable Assignment
0:29 Delete multiple items in List
0:48 Swapping Variables
▬▬▬▬▬▬ ⭐️ Follow me ⭐️ ▬▬▬▬▬▬
Linkedin - [ Ссылка ]
Twitter - [ Ссылка ]
To learn more on DevOps visit - [ Ссылка ]
Disclaimer/Policy: All the content/instructions are solely mine. The source is completely open-source.
Video is copyrighted and can not be re-distributed on any platform.
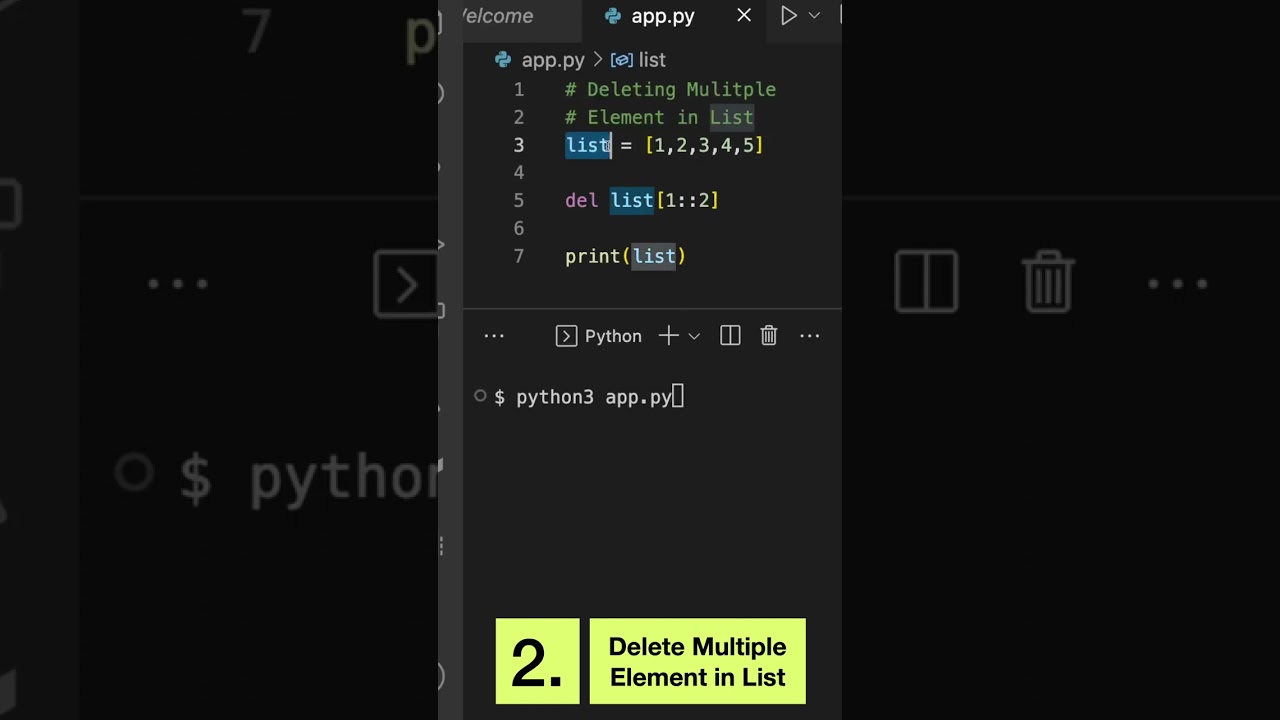