In Flutter, const and final are two keywords that are often confused with each other. While they both seem to imply that a variable's value cannot be changed, there are key differences between them:
# Const
- const is used to declare compile-time constants.
- The value of a const variable must be known at compile-time.
- const variables are implicitly final, meaning their value cannot be changed once set.
- const variables are evaluated at compile-time, which can improve performance.
# Final
- final is used to declare runtime constants.
- The value of a final variable can be determined at runtime.
- final variables can be reassigned, but only once.
- final variables are not evaluated until runtime.
Key differences:
- Timing: const values are determined at compile-time, while final values are determined at runtime.
- Immutability: Both const and final variables are immutable, but const variables are more restrictive since their values must be known at compile-time.
- Performance: const variables can improve performance since their values are evaluated at compile-time.
Example:
// Const example
const int compileTimeConstant = 10;
// Final example
final int runtimeConstant = DateTime.now().millisecondsSinceEpoch;
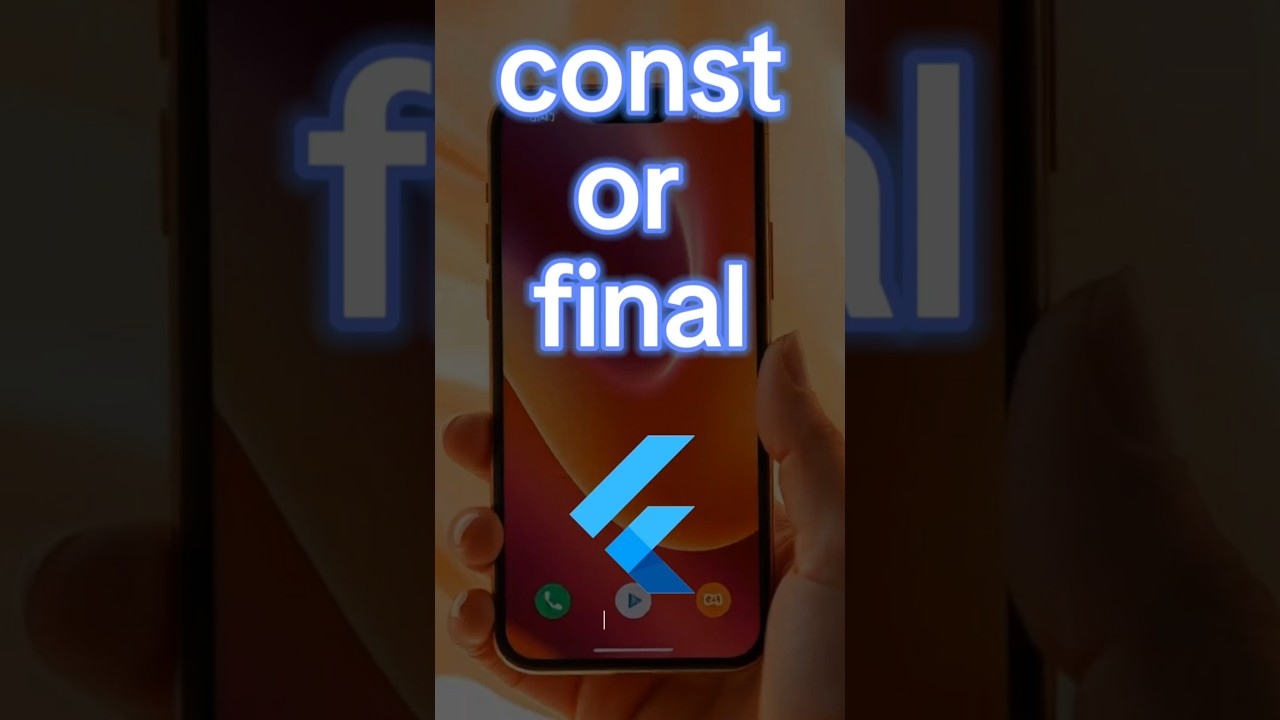