All FREE courses - [ Ссылка ]
Selenium Quiz - [ Ссылка ]
GitHub - [ Ссылка ]
00:00 Introduction
01:35 Selenium 4
03:48 Getting Started - Project Setup
09:31 1st Test
19:30 WebDriverManager
24:33 Browser Actions
25:25 How to open a web page
26:23 Get current URL
27:00 Get Title
27:53 Forward | Back | Refresh
29:44 Switching windows
32:12 Open new Window
33:26 Open new Tab
36:58 Close Browser
38:00 Frames
44:20 Get & Set Window size
48:49 Get & Set Window position
51:44 Maximize | Minimize | Full Screen
53:06 Screenshot
59:04 JavaScript Executor
01:03:58 Conclusion
Selenium 4
--------------
New and improved version of Selenium
Se webdriver can directly communicate with browser using W3C protocol
(without use of json wire protocol as earlier)
New functions added
Multiple windows & tabs management
Relative locators
New Documentation
Getting Started
-------------------
Install Java
Setup Eclipse
Create a new maven project
Add maven dependencies for Selenium 4
Download browser driver & add in a folder in project
(Can also keep at any location on your system and add the location in path env var)
Optional
Add TestNG plugin
Add TestNG dependency
1st Test
---------
Step 1 - Create a class & main method
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "./driver/chromedriver.exe");
WebDriver driver = new ChromeDriver();
//timeout driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10)); driver.manage().timeouts().scriptTimeout(Duration.ofMinutes(2)); driver.manage().timeouts().pageLoadTimeout(Duration.ofSeconds(10));
//close
driver.close();
}
Step 2 - Run code
Using WebDriver Manager
Step 1 - Add maven dependency for webdriver manager -
[ Ссылка ]
Step 2 - Add code
WebDriverManager.chromedriver().setup();
Step 3 - To use specific ver of browser
WebDriverManager.chromedriver().driverVersion("92.0").setup();
Browser Actions
----------------------
1. Open a web page
driver.get("[ Ссылка ]");
driver.navigate().to("[ Ссылка ]");
2. Get current url
driver.getCurrentUrl();
3. Get title
driver.getTitle();
4. Forward | Back | Refresh
driver.navigate().back();
driver.navigate().forward();
driver.navigate().refresh();
5. Switching windows
String originalWindow = driver.getWindowHandle();
driver.switchTo().window(originalWindow);
6. Open new window and switch to the window
driver.switchTo().newWindow(WindowType.WINDOW);
7. Open new tab and switch to the tab
driver.switchTo().newWindow(WindowType.TAB);
8. Closing browser
driver.close();
driver.quit();
9. Frames
Locate and Switch
WebElement iframe = driver.findElement(By.cssSelector(".rightContainer>frame"));
driver.switchTo().frame(iframe);
Using id or name
driver.switchTo().frame("classFrame");
Using index
driver.switchTo().frame(1);
Return to top level window
driver.switchTo().defaultContent();
10. Window management - Size
Get width & height
int width = driver.manage().window().getSize().getWidth();
int height = driver.manage().window().getSize().getHeight();
Store dimensions & query later
Dimension size = driver.manage().window().getSize();
int width1 = size.getWidth();
int height1 = size.getHeight();
Set window size
driver.manage().window().setSize(new Dimension(800, 600));
10. Window management - Position
Access x and y dimensions individually
int x = driver.manage().window().getPosition().getX();
int y = driver.manage().window().getPosition().getY();
Store dimensions & query later
Point position = driver.manage().window().getPosition();
int x1 = position.getX();
int y1 = position.getY();
Move the window to the top left of the primary monitor
driver.manage().window().setPosition(new Point(0, 0));
10. Window management
// maximize window
driver.manage().window().maximize();
// minimize window
driver.manage().window().minimize();
// fullscreen
driver.manage().window().fullscreen();
11. Screenshots
Take screenshot
File scrFile = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(scrFile, new File("./image.png"));
Take element screenshot
WebElement element = driver.findElement(By.cssSelector(".lnXdpd"));
File scrFile1 = element.getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(scrFile1, new File("./image1.png"));
12. JavaScript
Create JavascriptExecutor interface object by Type casting
JavascriptExecutor js = (JavascriptExecutor)driver;
Get return value from script
WebElement button =driver.findElement(By.name("btnI"));
String text = (String) js.executeScript("return arguments[0].innerText", button);
JavaScript to click element
js.executeScript("arguments[0].click();", button);
Execute JS directly
js.executeScript("console.log('hello world')");
#Selenium4Tutorials
If my work has helped you, consider helping any animal near you, in any way you can.
Never Stop Learning
Raghav
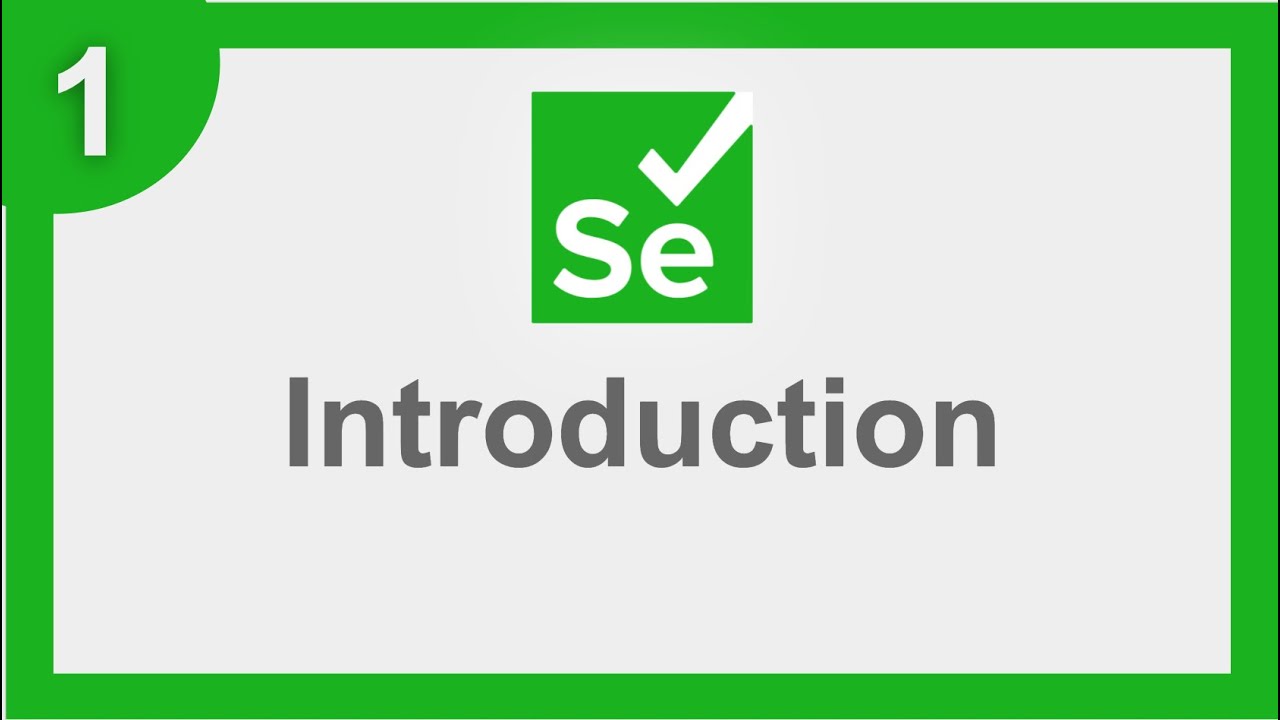