SELENIUM : How do you handle multiple windows using Selenium WebDriver in Java?
SDET Automation Testing Interview Questions & Answers
We will be covering a wide range of topics including QA manual testing, automation testing, Selenium, Java, Jenkins, Cucumber, Maven, and various testing frameworks.
SELENIUM : How do you handle multiple windows using Selenium WebDriver in Java?
To handle multiple windows using Selenium WebDriver in Java, you can use the getWindowHandles() method and the switchTo() method.
The getWindowHandles() method returns a set of handles to all open windows, and the switchTo() method can be used to switch the driver's focus to a specific window.
import java.util.Set;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class MultipleWindows {
public static void main(String[] args) {
// Create a new instance of the ChromeDriver
WebDriver driver = new ChromeDriver();
// Navigate to the page with the links to open new windows
driver.get("[ Ссылка ]");
// Find the link to open a new window
WebElement link = driver.findElement(By.id("link-id"));
// Click the link to open a new window
link.click();
// Get the handles to all open windows
Set handles = driver.getWindowHandles();
// Switch the driver's focus to the new window
for (String handle : handles) {
driver.switchTo().window(handle);
}
// Perform actions in the new window
// Close the new window
driver.close();
// Switch the driver's focus back to the original window
driver.switchTo().window((String) handles.toArray()[0]);
// Quit the driver
driver.quit();
}
}
This code navigates to a page, finds a link using the findElement() method, clicks the link to open a new window, gets the handles to all open windows using the getWindowHandles() method, switches the driver's focus to the new window using a for loop and the switchTo().window() method, performs actions in the new window, closes the new window using the close() method, switches the driver's focus back to the original window using the switchTo().window() method, and quits the driver using the quit() method.
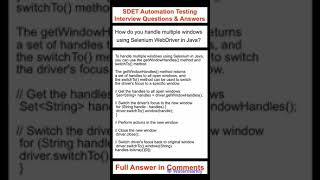