While loop statements - Repetition control structure - Java programming tutorial for beginners
Hi and welcome to this channel,
In today’s video, we will start our discussion on Repetition control structures.
Sometimes when writing a program you would want to repeat a set of statements a bunch of times.
One way you can do that is by typing the statements in the program over and over again.
This way of doing or this approach is ineffective or even impossible especially when you have huge amounts of statements to type.
That’s exactly the reason why we need to learn about repetition control structures. Because they provide a simpler approach when we want to repeat a set of statements in our code.
Repetition structures are also called Looping structures and in Java, there are three types of looping structures which are the while loop, the for loop, and the do … while loop.
In this video, we will start discussing the while loop structure
Here is the syntax of a while loop statement
int score = 0;
while (score less than or equal to 20)
System.out.println(i + “ “);
while, here is a reserved word
Following, in the brackets, is the logical expression also called a loop condition or simply a condition
Next, we have the action statements which is also called the body of the loop
The action statements are executed only when the loop condition is met
The action statement can be either a simple statement meaning that you will have only one statement
Or a compound statement when you have more than one statement
In case of a compound statement, you always need to surround your action statements with curly braces like this
int score = 0
while (score less than or equal to 20)
System.out.println(i + “ “);
score = score + 5;
Here is what will happen when we run this portion of code
First of all, note that we have declared and initialized an integer variable score. This variable is called the Loop control variable.
Next, the logical expression in the while loop statement is then evaluated.
Because the condition score less than or equal to 20 evaluates to true, then the action statements in the body of the loop will execute
The first action statement will output the value of score
While the second action statement will increment the value of the variable score by 5
After, the logical expression in the while loop is evaluated again
Because the value of the variable score is now 5 the logical expression score less than or equal to 20 will still evaluate to true and the body of the while loop will execute
By outputting the values of the variable score
And incrementing the value of the variable score by 5 again
This process of evaluating the logical expression and executing the body of the while loop will continue until the expression score less than or equal to 20 evaluates to false
That’s the reason why we are getting in the console
0 5 10 15 20
There will come a point in the loop, when the value of the variable score will become 25
But this will not be printed out
Simply because, when the variable score becomes 25 the condition score than or equal to 20 evaluates to false
Based on this example there are some very important point we have to note about while loops
The first point to note is that you must always initialize the loop control variable, score, before you execute the loop
If you remove the initialization int score = 0
Notice that the compiler is generating an error
Telling us to initialize the variable
The second point is that if you forget the second action statement score = score + 5 in the body of the loop
You will have an infinite loop
An infinite loop is a loop that continues to execute endlessly
Let me remove the statement score = score + 5
And run
See that our loop is executing endlessly
In order to avoid an infinite loop, you always have to make sure that the loop’s body contains a statement that ensures that the condition in the while loop will eventually evaluate to false at a certain moment
In our case, the statement score = score + 5 does just that
The third point to note also, is that interchanging the sequence of the statements in the body of the loop may alter the result of the while loop
For example, if I put the statement score = score + 5 before the output statement, like this
int score = 0
while (score less than or equal to 20)
score = score + 5;
System.out.println(i + “ “);
And run this code
We will get
5 10 15 20 25
Printed in the console
Typically, this is considered to be a semantic error because you would not want a condition to be true for score less than or equal to 20, and yet produce results for score greater than 20
Thanks for viewing, I hope this video was informative.
Please do not forget to like and SUBSCRIBE to this channel.
Let’s meet in the next video
#codingriver
#java
#programming
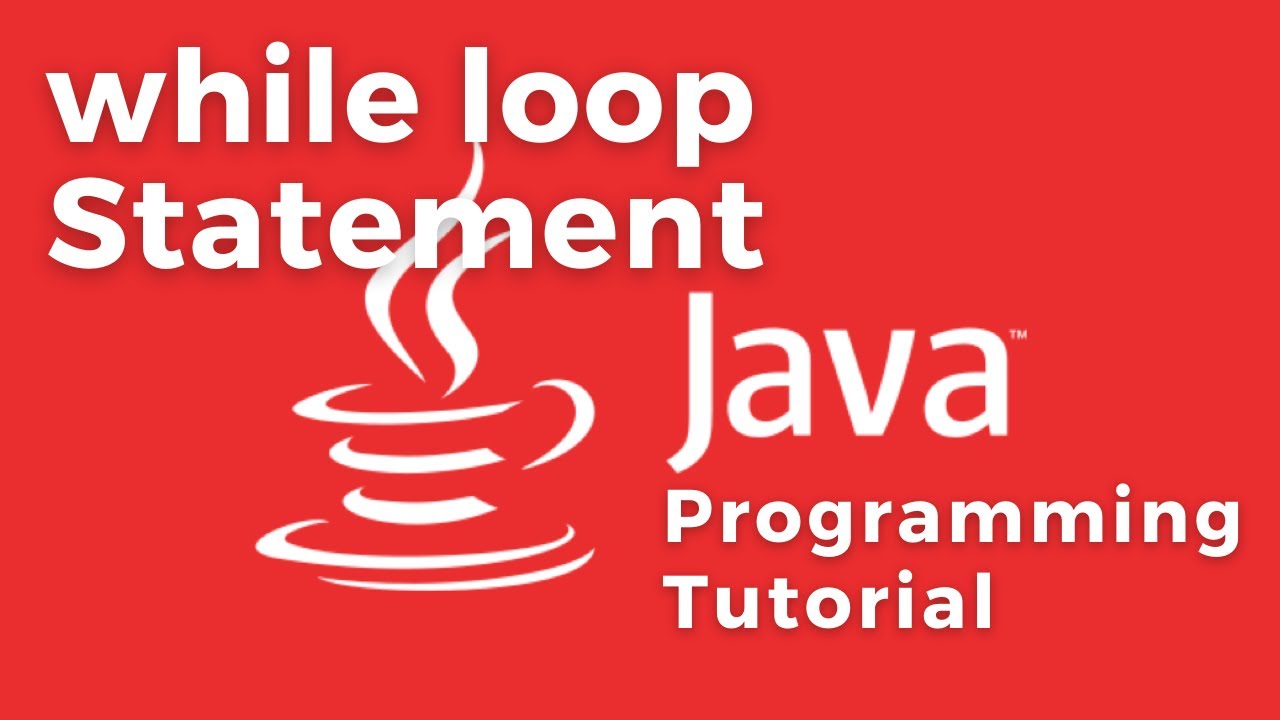