Learn how to create and implement a delegate for NSUserNotificationCenter in Swift to handle notifications more effectively.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
How to Create a Delegate for NSUserNotificationCenter in Swift
Delegation is a core pattern in Swift that facilitates object-to-object communication, often used to handle specific tasks. In this post, we will focus on creating a delegate for NSUserNotificationCenter in Swift, a key framework for managing and triggering local notifications on macOS.
What is a Delegate in Swift?
A delegate in Swift is a design pattern that allows one object to communicate with another and act on its behalf. This pattern is typically used to manage complex operations without creating tight couplings between objects. A delegate object implements a protocol defined by the delegating object, thereby allowing the latter to request or notify the former to act upon specific events or data.
Creating a Delegate for NSUserNotificationCenter
NSUserNotificationCenter is responsible for delivering and managing notifications in macOS applications. Setting a delegate allows you to handle user interactions and customize notification behaviors.
Step-by-Step Guide:
Declare the Delegate Protocol:
To begin, create a class that conforms to the NSUserNotificationCenterDelegate protocol. This will involve implementing various delegate methods defined in the protocol.
[[See Video to Reveal this Text or Code Snippet]]
Assign the Delegate:
After defining your delegate class, assign an instance of this class to the delegate property of NSUserNotificationCenter.
[[See Video to Reveal this Text or Code Snippet]]
Creating and Scheduling a Notification:
Create a simple notification and schedule it using NSUserNotificationCenter.
[[See Video to Reveal this Text or Code Snippet]]
Handling Notification Actions:
Implement actions based on the user’s interaction with the notification, as defined in your delegate methods.
Conclusion
By creating a delegate for NSUserNotificationCenter, you can customize the behavior of notifications and handle user interactions more effectively. Adopting the delegation pattern in Swift not only promotes clean code architecture but also enhances modularity and reusability within your app.
Delegation is a powerful design pattern in Swift, offering robust and flexible solutions for managing complex object interactions with minimal coupling between components.
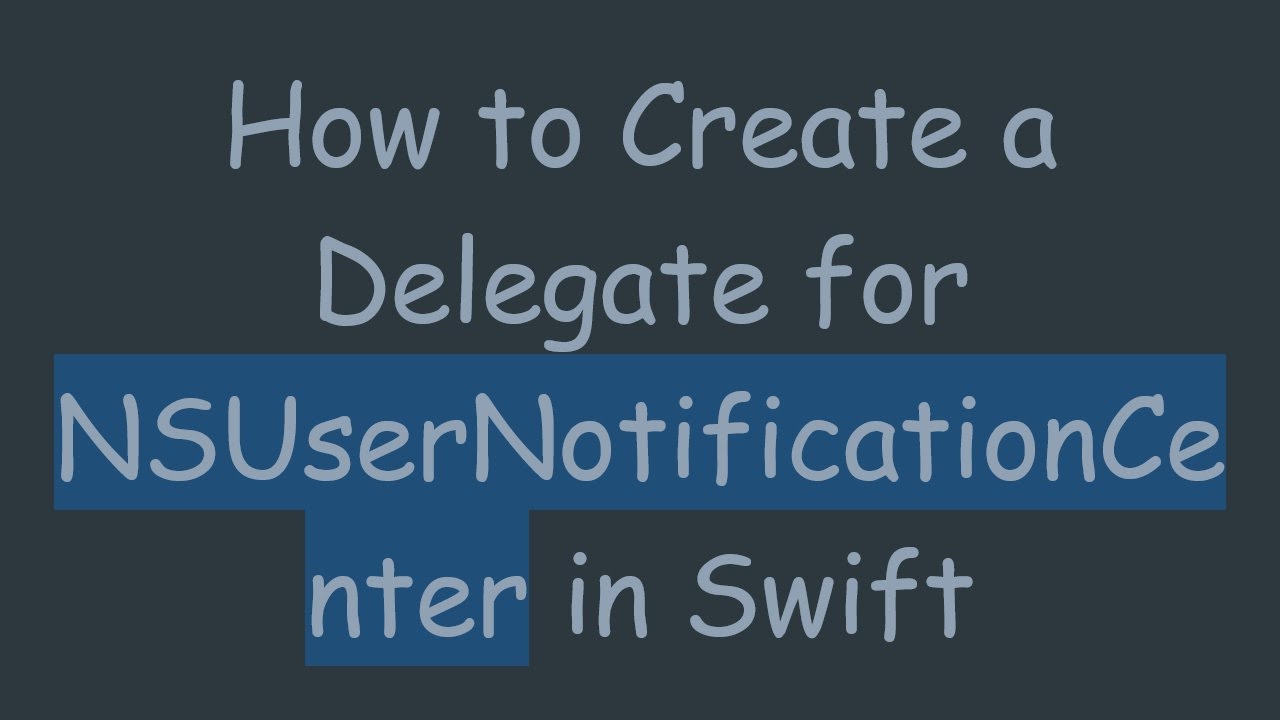