#dharasinfotech
Function declaration A function must be declared globally in a c program to tell the compiler about the function name, function parameters, and return type. Function call Function can be called from anywhere in the program.
Functions with arguments and return values. This function has arguments and returns a value: ...
Functions with arguments and without return values. ...
Functions without arguments and with return values. ...
Functions without arguments and without return values.
The syntax for creating a function is as follows: Here, the return type is the data type of the value that the function will return. Then there is the function name, followed by the parameters which are not mandatory, which means a function may or may not contain parameters.
A function is a block of code that performs a specific task.
Suppose, you need to create a program to create a circle and color it. You can create two functions to solve this problem:
create a circle function
create a color function
Dividing a complex problem into smaller chunks makes our program easy to understand and reuse.
Standard library functions
The standard library functions are built-in functions in C programming.
These functions are defined in header files. For example,
The printf() is a standard library function to send formatted output to the screen (display output on the screen). This function is defined in the stdio.h header file.
Hence, to use the printf()function, we need to include the stdio.h header file using #include stdio.h.
The sqrt() function calculates the square root of a number. The function is defined in the math.h header file.
Note, function names are identifiers and should be unique.
This is just an overview of user-defined functions. Visit these pages to learn more on:
User-defined Function in C programming
Functions in C are the basic building blocks of a C program. A function is a set of statements enclosed within curly brackets ({}) that take inputs, do the computation, and provide the resultant output. You can call a function multiple times, thereby allowing reusability and modularity in C programming. It means that instead of writing the same code again and again for different arguments, you can simply enclose the code and make it a function and then call it multiple times by merely passing the various arguments.
Why Do We Need Functions in C Programming?
We need functions in C programming and even in other programming languages due to the numerous advantages they provide to the developer. Some of the key benefits of using functions are:
Enables reusability and reduces redundancy
Makes a code modular
Provides abstraction functionality
The program becomes easy to understand and manage
Breaks an extensive program into smaller and simpler pieces
Basic Syntax of Functions
The basic syntax of functions in C programming is:
return_type function_name(arg1, arg2, … argn){
Body of the function //Statements to be processed
}
In the above syntax:
return_type: Here, we declare the data type of the value returned by functions. However, not all functions return a value. In such cases, the keyword void indicates to the compiler that the function will not return any value.
function_name: This is the function’s name that helps the compiler identify it whenever we call it.
arg1, arg2, ...argn: It is the argument or parameter list that contains all the parameters to be passed into the function. The list defines the data type, sequence, and the number of parameters to be passed to the function. A function may or may not require parameters. Hence, the parameter list is optional.
Body: The function’s body contains all the statements to be processed and executed whenever the function is called.
Types of user-defined Functions
Advantages of user-defined function
The program will be easier to understand, maintain and debug.
Reusable codes that can be used in other programs
A large program can be divided into smaller modules. Hence, a large project can be divided among many programmers.
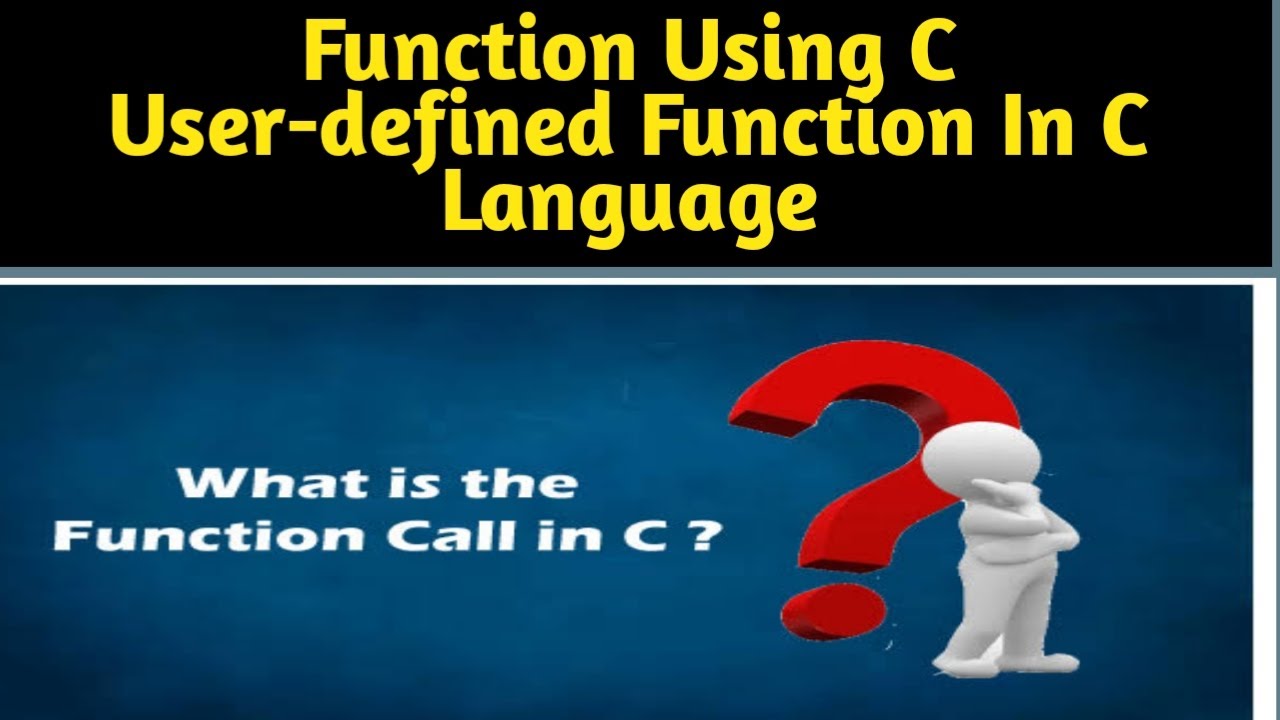