Learn how to effortlessly create a Python dictionary from multiple lists to manage your data more effectively.
---
Disclaimer/Disclosure - Portions of this content were created using Generative AI tools, which may result in inaccuracies or misleading information in the video. Please keep this in mind before making any decisions or taking any actions based on the content. If you have any concerns, don't hesitate to leave a comment. Thanks.
---
How to Create a Python Dictionary from Multiple Lists
If you are working with Python, you might find yourself in situations where you need to create a dictionary from multiple lists. This can be an efficient way to combine related data and utilize the powerful capabilities of dictionaries in Python. This guide will guide you through the process.
The Basics of Python Dictionary
A dictionary in Python is a collection of key-value pairs. It's a versatile data structure that allows you to store and access data efficiently. Dictionaries are created using curly braces {} or the dict() constructor.
[[See Video to Reveal this Text or Code Snippet]]
Creating a Dictionary from Multiple Lists
Assume you have two lists, one containing keys and the other containing values:
[[See Video to Reveal this Text or Code Snippet]]
Using the zip Function
One of the most efficient ways to create a dictionary from these lists is using the zip function, which pairs elements from two lists together, and then passing these pairs to the dict constructor.
[[See Video to Reveal this Text or Code Snippet]]
The output will be:
[[See Video to Reveal this Text or Code Snippet]]
Using Dictionary Comprehension
Another method is using dictionary comprehension. This method is slightly more verbose but can be more flexible when performing additional operations during the dictionary creation.
[[See Video to Reveal this Text or Code Snippet]]
This will produce the same output:
[[See Video to Reveal this Text or Code Snippet]]
Handling Unequal Length Lists
In real-world applications, lists might not always be of the same length. Here’s how you can handle such scenarios gracefully:
Truncating to the Shortest List
When you use zip, it automatically truncates the result to the length of the shortest list:
[[See Video to Reveal this Text or Code Snippet]]
Output:
[[See Video to Reveal this Text or Code Snippet]]
Filling with None or Defaults
If you want to ensure all keys have a value, you can use the itertools.zip_longest function:
[[See Video to Reveal this Text or Code Snippet]]
Output:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Creating a dictionary from multiple lists in Python can be straightforward and efficient with the right tools. The zip function and dictionary comprehensions offer versatile ways to achieve this, accommodating various data scenarios. By mastering these techniques, you can streamline your data management processes in Python.
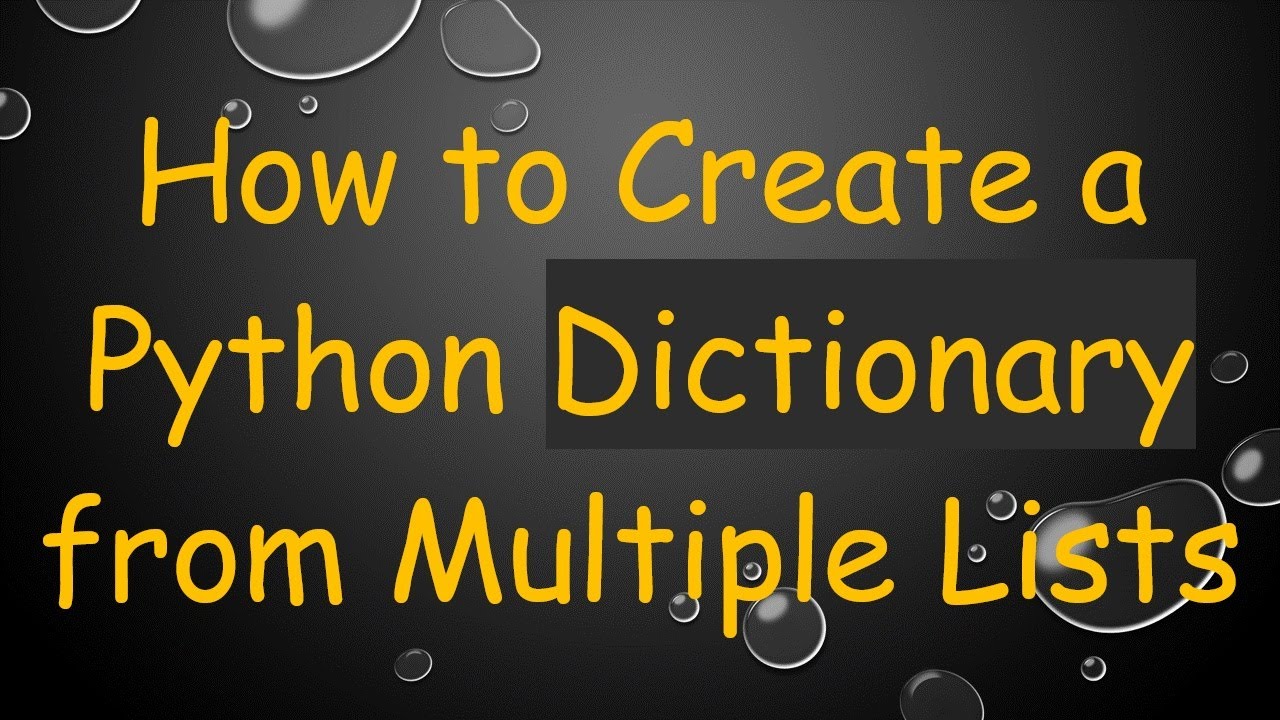