Learn how to create a custom exception class in Java specifically designed to handle scenarios where an integer array is empty.
---
Creating a Custom Exception Class in Java for an Empty Integer Array
In Java, exceptions are crucial for handling errors and other exceptional events that disrupt the normal flow of a program. While Java provides a range of built-in exceptions, sometimes you might need to create your own custom exceptions to handle specific scenarios more precisely. One such scenario could be the handling of an empty integer array. This guide will guide you through the creation of a custom exception class in Java to manage this specific condition.
Why Create a Custom Exception?
Built-in exceptions cover a wide range of situations, but custom exceptions can make your code more readable and specific. For example, if an integer array in your program should never be empty, a custom exception can communicate this clear requirement when the rule is violated.
Step-by-Step Guide to Creating a Custom Exception
Step 1: Define the Custom Exception Class
First, you need to define the custom exception class. Custom exceptions in Java are created by extending the Exception class or any of its subclasses. For our scenario, let's call our custom exception EmptyArrayException.
[[See Video to Reveal this Text or Code Snippet]]
In this example, EmptyArrayException extends the Exception class. The constructor takes a message as an argument, which will be used to describe the exceptional event.
Step 2: Throw the Custom Exception
Next, you'll need to throw this custom exception when an integer array is found to be empty in your code.
[[See Video to Reveal this Text or Code Snippet]]
In the processArray method, we check whether the array is null or its length is 0. If either condition is met, it throws our custom EmptyArrayException.
Step 3: Handle the Custom Exception
Lastly, wherever you invoke the method that can throw this custom exception, you must handle it appropriately, usually with a try-catch block.
[[See Video to Reveal this Text or Code Snippet]]
In the main method, we attempt to process an empty array. When the EmptyArrayException is thrown, it’s caught and handled by printing the message.
Conclusion
Creating a custom exception, like EmptyArrayException, allows for more precise error handling and makes your code more readable and maintainable. By following the steps outlined in this post, you can create and use custom exceptions to handle specific conditions in your Java programs effectively.
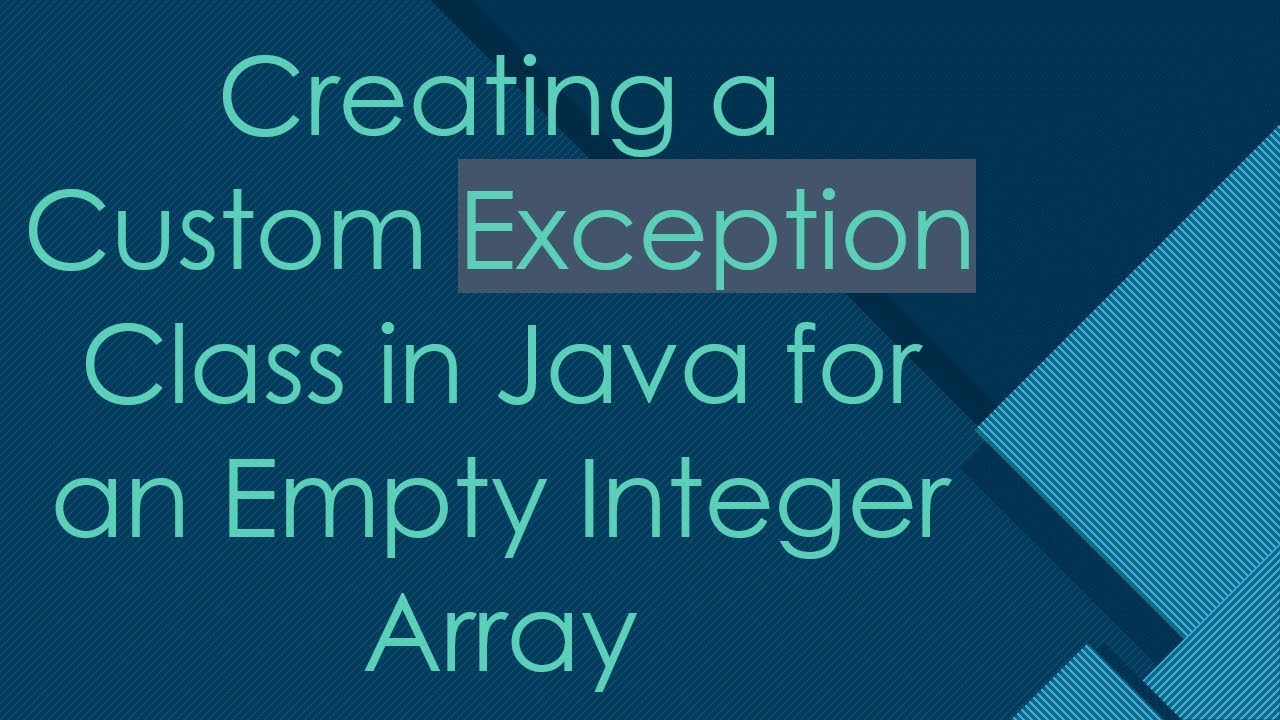