Summary: Explore the common error "subprocess check_output returned non zero exit status 1" in Python, understand its causes, and learn effective ways to handle it.
---
Understanding 'subprocess check_output returned non zero exit status 1' Error in Python
Working with the Python subprocess module can be quite useful when you need to execute shell commands or interact with external programs directly within your Python script. However, one of the errors that can stump developers during this process is:
[[See Video to Reveal this Text or Code Snippet]]
In this guide, we’ll discuss what this error means, why it happens, and how you can handle it effectively in your Python code.
What Does the Error Indicate?
When you encounter the error subprocess check_output returned non zero exit status 1, it essentially means that the command you attempted to execute via subprocess.check_output() has failed. To be more specific:
subprocess.check_output(): This function runs a command with arguments and returns its output. If the command returns a non-zero exit status, a CalledProcessError exception is raised.
Non-zero exit status: In Unix-like operating systems, an exit status of 0 indicates success. Any non-zero exit status, such as 1, indicates that an error occurred.
Common Causes
Several factors can lead to this error:
Invalid Command or Arguments: The command or the arguments passed to it might be incorrect or misspelled.
Permissions Issues: The command might require administrative privileges that the script does not have.
Environment Variables: Necessary environment variables might not be set correctly in the environment where the script is executed.
Dependencies: External dependencies or resources that the command relies on might be missing or incompatible.
Internal Program Errors: The program that you are calling might contain errors causing it to exit with a non-zero status.
How to Handle the Error
Handling this error gracefully involves the following steps:
Verify the Command and Arguments
Before anything else, ensure that the command and its arguments are correct and functional by testing them directly in the terminal.
Use Try-Except Block
Catch the CalledProcessError exception to handle the error gracefully.
[[See Video to Reveal this Text or Code Snippet]]
Check Permissions
Make sure that the user running the script has the necessary permissions to execute the command. You may need to run the script with elevated privileges using sudo.
[[See Video to Reveal this Text or Code Snippet]]
Set Environment Variables
Ensure that any required environment variables are set properly before running the command.
[[See Video to Reveal this Text or Code Snippet]]
Validate External Dependencies
Ensure all external dependencies and resources are correctly installed and compatible with your system.
Conclusion
The subprocess check_output returned non zero exit status 1 error can be a bit daunting at first glance, but it’s typically straightforward to diagnose and fix. By carefully checking the command you’re running, catching exceptions, and ensuring your environment is configured correctly, you can often resolve this error without much hassle. Happy coding!
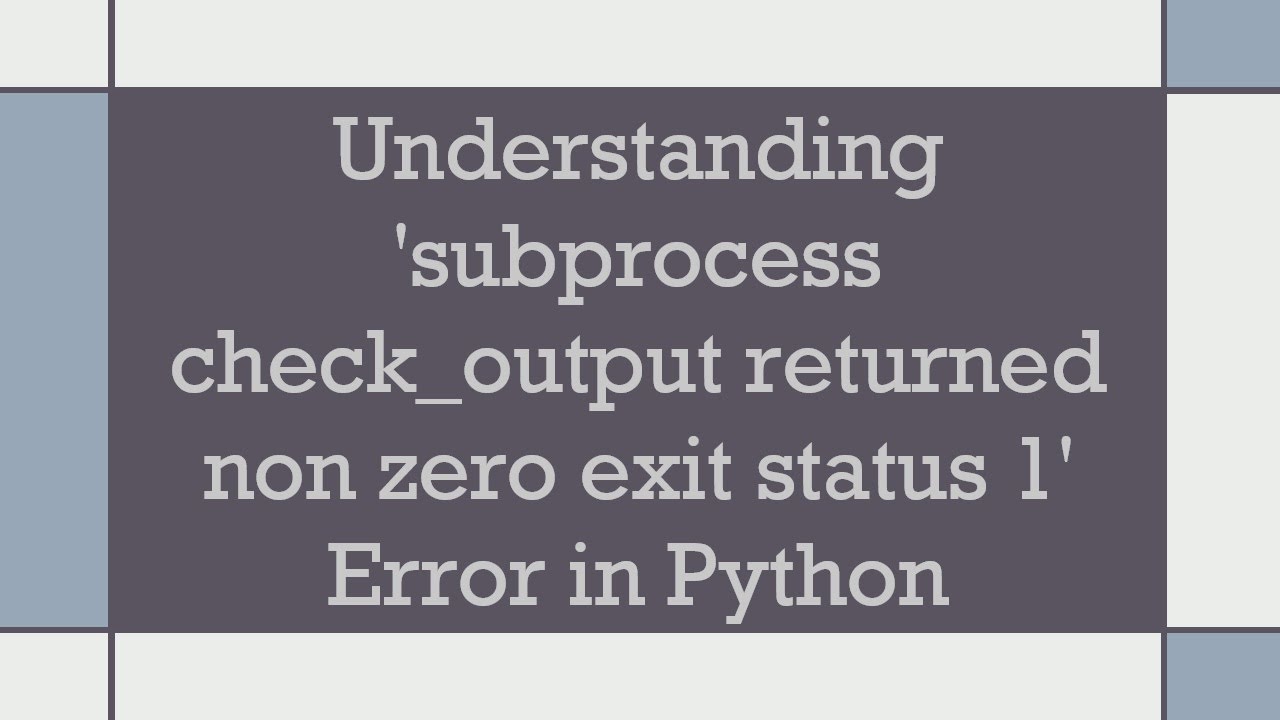